Table Of Content
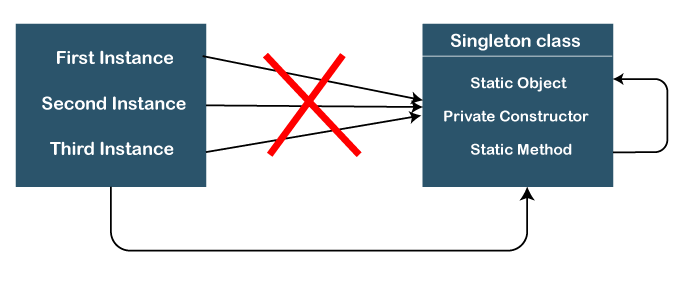
The necessary elements of a C++ class are constructors and destructors.By default these are generated by the compiler, and public. The Logger should have a print method taking as input a log level (INFO, WARN, ERROR), and a message. In the next chapter I will explain about one of the most used design pattern named as Singleton design pattern.
Design patterns are typical solutions to commonly occurring problems in software design. They are like pre-made…
The new variable also points to the initial instance created if we attempt to instantiate the Singleton class after the first time. This is implemented by using the core concepts of object-oriented programming namely access modifiers, constructors & static methods. The implementation of the singleton Design pattern is very simple and consists of a single class.
thoughts on “Singleton Design Pattern in C#”
All subsequent calls to the GetInstance method return the same instance. A singleton implementation may use lazy initialization in which the instance is created when the static method is first invoked. In multithreaded programs, this can cause race conditions that result in the creation of multiple instances. The following Java 5+ example[6] is a thread-safe implementation, using lazy initialization with double-checked locking. The Singleton Design Pattern is one of the creational design patterns used in software engineering.
Related Articles
These different objects try to invoke an object instantiated as a singleton called Singleton Obj, and That single instance of the object helps us to invoke underneath methods or events. In this article, we learn about Design Patterns, the evolution of Design Patterns, and the three types of design patterns. It is important to know about the interfaces, abstract classes, delegates, and other features related to the Oops concept, along with the design pattern. For understanding design patterns, It is very important to have knowledge about the below object-oriented concepts. Design patterns is the important features of object oriented programming.
Sign up or log in
Let's highlight the advantages of singleton class or pattern first before we jump into actual implementation. In our case, our log file is the shared resource and singleton makes sure every client accesses it with no deadlock or conflict. The second advantage is that it only allows one instance of a class responsible for sharing the resource, which is shared across all the clients or applications in a controlled state. The Singleton design pattern is a fundamental concept in software engineering that ensures a class has only one instance while providing a global point of access to that instance.
This still isn't too big of a deal if you are relying on program termination to clean up anyway. Then, the Logger class and main application I built are very simplistic examples. The Internet is full of examples of more interesting applications. First of all, the limits of our program are set through global parameters.We will set a maximum number of threads, no need to go beyond 1000 threads. You can find on GitHub the final result of the Logger class header and implementation.
Main function
Implementing the Singleton Design Pattern in C# .NET can significantly enhance your application’s architecture and maintainability. By understanding its core principles and using it wisely, you can create more efficient, scalable, and organized software systems. Let’s dive into a practical example of implementing the Singleton pattern in C# .NET. Consider a scenario where we want to manage print jobs using a PrintSpooler class.

Depending on the specific application requirements, the Singleton pattern can be implemented with different variations, such as lazy/eager initialization, or additional thread safety measures. These variations may affect how the instance is created and accessed, but the fundamental concept of a single instance with global access remains unchanged. Also, because of its disadvantages, especially the violations of some SOLID principles and the tight coupling, the Singleton pattern is considered an Anti-Pattern. Here, we created the Singleton class as sealed, which ensures that the class cannot be inherited from the derived classes.
Top 30 C# OOPS Interview Questions and Answers for 2024 - Simplilearn
Top 30 C# OOPS Interview Questions and Answers for 2024.
Posted: Mon, 26 Sep 2022 08:07:50 GMT [source]
Software Design Pattern in Development
85 Must-Know C# Interview Questions and Answers [2024] - Simplilearn
85 Must-Know C# Interview Questions and Answers .
Posted: Mon, 15 Apr 2024 07:00:00 GMT [source]
The above class creates an instance as soon as we access any static property or method. If there are multiple static properties or methods for some reason then an instance will be created immediately even if we don't intend to use it. We need lazy instantiation that will create instances only when necessary.
The class is created with a private parameterless constructor, ensuring that the class will not be instantiated from outside the class. Again, we declared the instance variable private and initialized it with the null value, ensuring that only one class instance will be created based on the null condition. The public method, GetInstance, returns only one instance of the class by checking the value of the private variable instance. The public method PrintDetails can be invoked from outside the class through the singleton instance. A singleton class is a special type of class in object-oriented programming which can have only one object or instance at a time. In other words, we can instantiate only one instance of the singleton class.
In 1994, 4 authors known as “Gang of Four” released a book named as “Design Patterns”. In that book they divided all these design patterns into three categories. At the moment there are 23 design patterns and all these are categorize under these 3 type of categories named as, Creational, Structural, and Behavioral. There is another way to give back the singleton object creation.
No comments:
Post a Comment